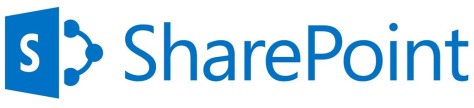
Collaborative Application Markup Language (CAML) is an XML-based language that is used in Microsoft SharePoint Foundation to define the fields and views that are used in sites and lists.
We can use queries to get data from SharePoint lists with filtering. As a best practice, it is recommended to filter data for large lists to improve performance.
Following can be various samples when we use CAML queries.
1) CAML query with simple filtering
SPQuery oquery = newSPQuery();
oquery.Query = “<Where><Eq><FieldRef Name=’Child_x0020_Type’ /><Value Type=’Text’>” + childType.ToString().Trim() + “</Value></Eq></Where>”;
2) CAML query with SORTING (OrderBy)
SPQuery oquery = newSPQuery();
oquery.Query = “<Where><Eq><FieldRef Name=’Article_ID’ /><Value Type=’Text’>” + articleID.ToString().Trim() + “</Value></Eq></Where><OrderBy><FieldRef Name =\”Modified\” Ascending=\”FALSE\”/></OrderBy>”;
SPListItemCollection col = lstTestArticles.GetItems(oquery);
3) CAML query with few conditions (AND, AND)
SPQuery oquery = newSPQuery();
oquery.Query = “<Where><And><And><Eq><FieldRef Name=’Child_x0020_Type’ /><Value Type=’Text’>” + childType.ToString().Trim() + “</Value></Eq>” +
“<Eq><FieldRef Name=’Parent_x0020_Type’ /><Value Type=’Text’>” + parentType + “</Value></Eq></And>” + “<Eq><FieldRef Name=’Article_x0020_Type’ /><Value Type=’Text’>” + articleType.ToString().Trim() + “</Value></Eq>” +
“</And></Where><OrderBy><FieldRef Name =’Title’ Ascending=’TRUE’/></OrderBy>”;
4) CAML query with DATETIME
SPQuery oquery = newSPQuery();
query.Query = “<Where><And><Geq><FieldRef Name=’Modified’ /><Value Type=’DateTime’ IncludeTimeValue=’TRUE’>” + SPUtility.CreateISO8601DateTimeFromSystemDateTime(dtStartTime.SelectedDate) + “</Value></Geq>” +
“<Leq><FieldRef Name=’Modified’ /><Value Type=’DateTime’ IncludeTimeValue=’TRUE’>” +
SPUtility.CreateISO8601DateTimeFromSystemDateTime(dtendTime.SelectedDate) + “</Value></Leq></And></Where>”;
5) CAML query with USER
SPQuery oquery = newSPQuery();
query.Query = “<Where><And><And><Geq><FieldRef Name=’Modified’ /><Value Type=’DateTime’ IncludeTimeValue=’TRUE’>” + SPUtility.CreateISO8601DateTimeFromSystemDateTime(dtStartTime.SelectedDate) + “</Value></Geq>” +
“<Leq><FieldRef Name=’Modified’ /><Value Type=’DateTime’ IncludeTimeValue=’TRUE’>” + SPUtility.CreateISO8601DateTimeFromSystemDateTime(dtendTime.SelectedDate) + “</Value></Leq></And><Eq><FieldRef Name=’Editor’ LookupId=’TRUE’ /><Value Type=’User’>” + myuser.ID + “</Value></Eq></And></Where>”;
6) CAML query with Integer
SPQuery oquery = newSPQuery();
oquery.Query = “<Where><And><Eq><FieldRef Name=’Article_x0020_Type’ /><Value Type=’Text’>” + articleCategory.ToString().Trim() + “</Value></Eq>” +
“<Eq><FieldRef Name=’Status’ /><Value Type=’Integer’>” + 1 + “</Value></Eq>” +
“</And></Where>”;
SPListItemCollection oCol = TestArticle.GetItems(oquery);
7) CAML Query to get documents
SPWeb oWeb = SPContext.Current.Web;
SPList olist = oWeb.Lists[Constants.TESTProcessRootFolderName()];
SPView oView = olist.Views[“All Documents”];
SPQuery oQuery = newSPQuery(oView);
oQuery.RowLimit = 22;
oQuery.ViewAttributes = “Scope=\”Recursive\””;
oQuery.Query = “<Where><Contains><FieldRef Name=’Title’ /><Value Type=’Text’>” + relatedID.ToString(“00″) + “-” + “</Value></Contains></Where><OrderBy><FieldRef Name =\”Modified\” Ascending=\”FALSE\”/></OrderBy>”;
SPListItemCollection collListItemsAvailable = olist.GetItems(oQuery);
return collListItemsAvailable;
26.228516
50.586050